Creating a RESTful Web Service in Rust: A Comprehensive Guide
Written on
Introduction to RESTful Services
It has been nearly three months since my last article, and I've truly missed discussing Rust! In this piece, we will delve into the straightforward process of developing a RESTful web service in Rust using the Rocket crate.
What is REST?
A web service is considered RESTful when it adheres to a specific set of architectural principles known as Representational State Transfer (REST). The REST architecture promotes a stateless, cacheable system built on a layered client-server model, ensuring a consistent interface between its components while optionally allowing for code on demand. These principles extend the foundational concepts of the World Wide Web (WWW) and are frequently associated with the HTTP protocol (for more on HTTP, click here).
Let’s get creative and develop a simple RESTful web service that converts dog years into human years! (In simple terms, one dog year is equivalent to seven human years.)
Building a Web Service with Rocket
Similar to various programming languages, Rust offers numerous crates to facilitate the creation of web services. Rocket is a popular Rust web framework designed to simplify the development of fast and secure web applications without compromising flexibility, usability, or type safety. Its appeal lies in its features (like asynchronous processing and embedded templating) and the quality of available tutorials.
To kick off our new project, we can create a new project using the command:
cargo new web_service
Next, we must update the dependencies section of our Cargo.toml file with the following line to include Rocket:
rocket = "0.5-rc.2"
(The latest version of Rocket can always be checked on the official Rocket website.)
Our web application will feature the following endpoints:
- The root (/) endpoint, which sends a welcome message.
- The dog (dog) endpoint, which accepts a number representing dog years as a parameter and responds with a message that provides the equivalent in human years.
The following source code can be found in the file src/main.rs:
// Code snippet here
In this section of code, we define two route handler functions corresponding to each endpoint:
- The index function returns a welcome message to the client. This function is associated with a GET request made to the root (/) via the #[get('/')] attribute.
- The dog_years_to_human_years function takes the dog_years URL parameter, converts it to human years, and returns that information if the provided parameter is a positive number. Otherwise, it returns a warning message. This function is linked with the #[get('/dog/<dog_years>')] attribute, allowing Rocket to invoke it when a GET request is made to the /dog endpoint.
Additionally, we have the rocket function, which manages the setup and initialization of our Rocket web service. To ensure global access to the macros in the Rocket crate, we need to include the following line:
#[macro_use] extern crate rocket;
You can access the complete source code for this project on GitHub. The project can be launched with the command:
cargo run
This command will start the web server, and you will see a confirmation message in the CLI, indicating that the server has been successfully started.
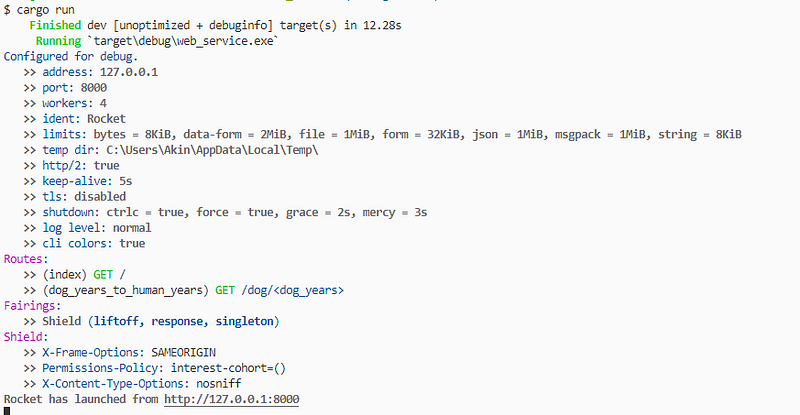
We did it! Our web service is now operational on port 8000. You can visit both endpoints:
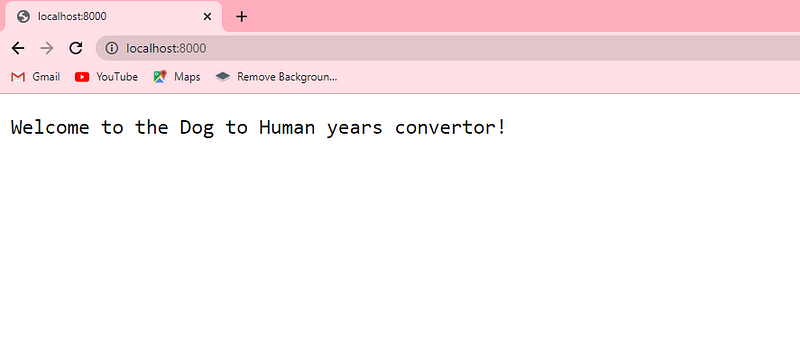
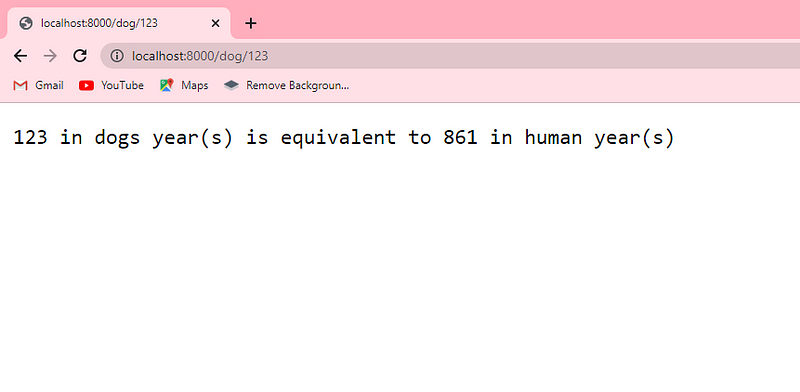
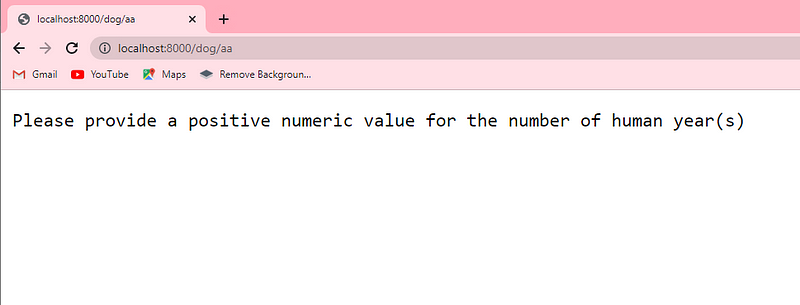
If you attempt to access an undefined endpoint, Rocket will handle it gracefully, as demonstrated below:
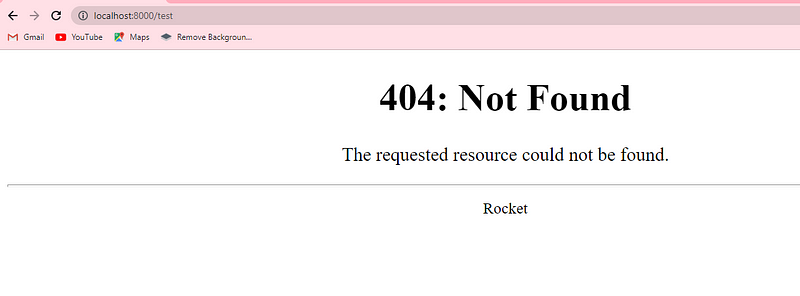
In summary, we have successfully built a simple web application that converts dog years into human years using Rust in no time at all.
Conclusion
Rocket is an exceptional Rust crate that enables rapid web service creation and provides a range of impressive built-in features. I plan to explore the templating and async functionalities in greater detail in future articles. As always, your feedback is appreciated; likes and claps are even better!
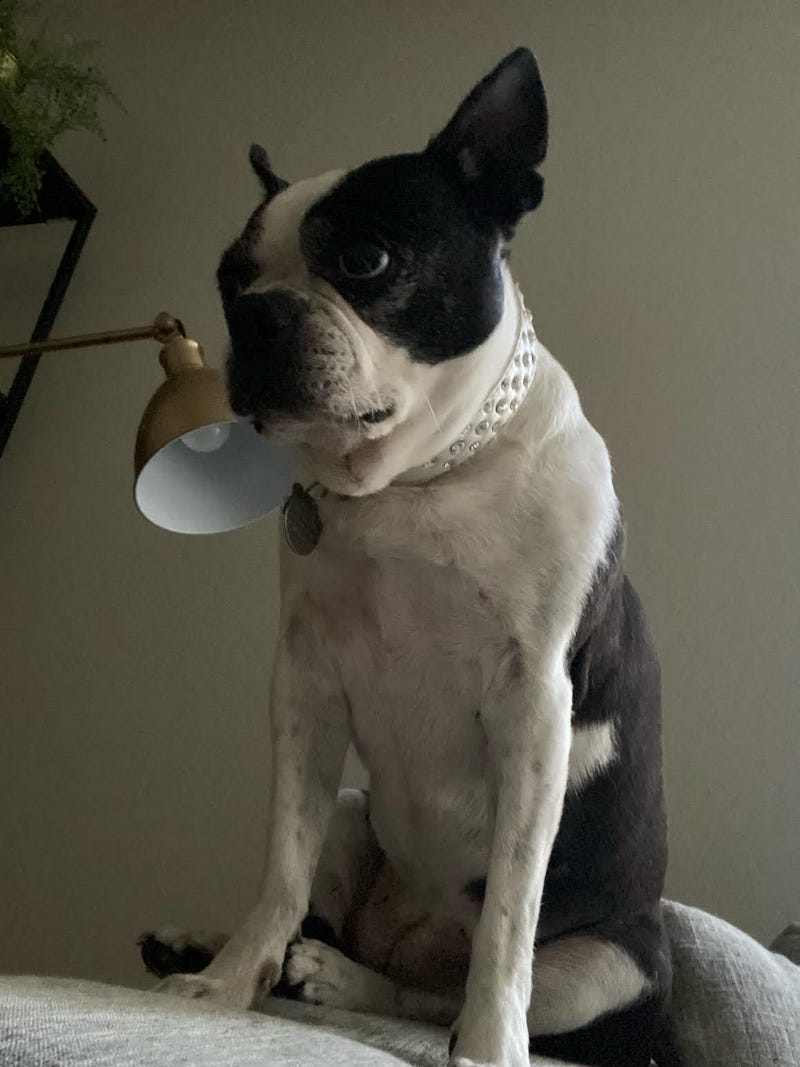
In this video titled "Rust Web Services: High-Performance REST API with Axum", you will discover how to leverage Axum for building high-performance REST APIs in Rust.
This video, "Building and Deploying a REST API in Rust | ShuttleBytes #1", provides insights on how to effectively build and deploy REST APIs using Rust.