Mastering JavaScript Basics for Interview Success: Day 7
Written on
Chapter 1: Introduction to Day 7
Welcome to Day 7 of our journey through 100 Days of JavaScript Interview Preparation. Today, we’ll dive deeper into the most frequently asked string manipulation questions in interviews. This series aims to enhance your skills through consistent practice and learning.
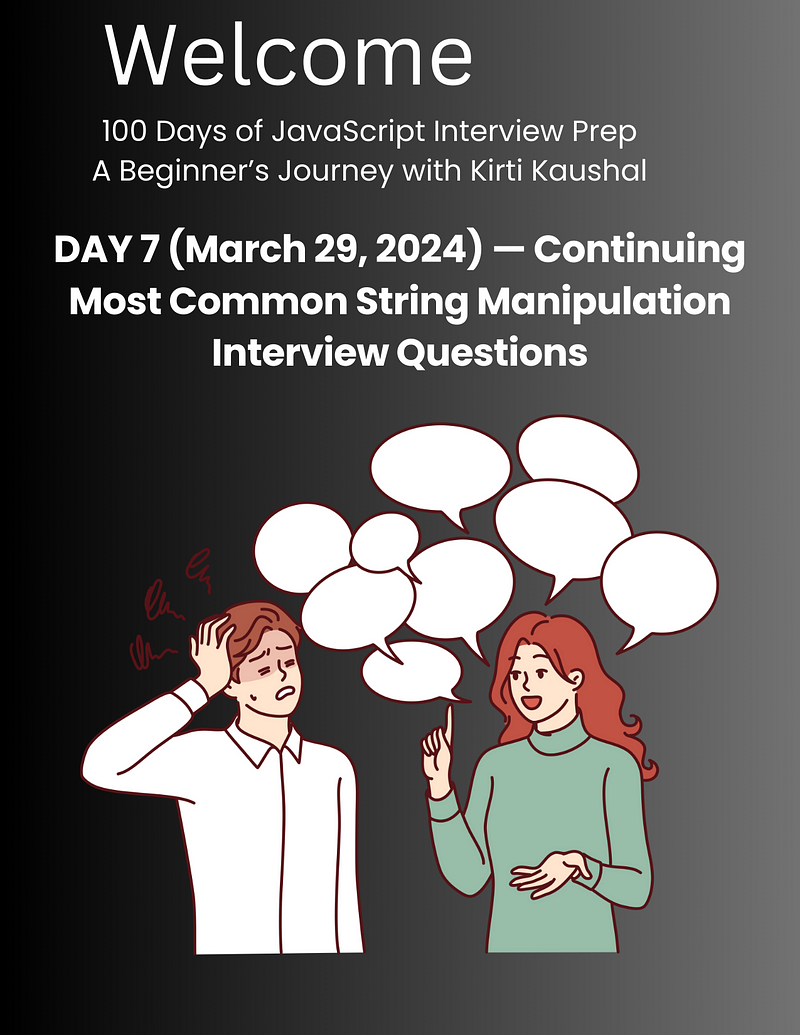
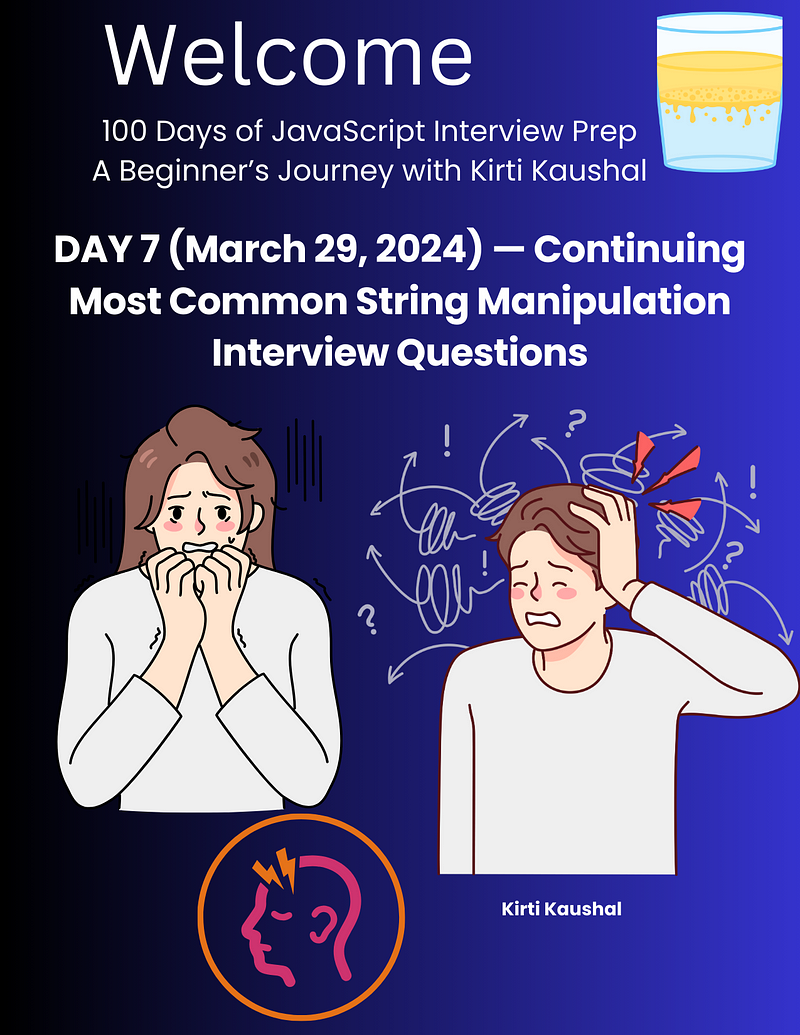
DAY 7 (March 29, 2024) — Continuing with Common String Manipulation Questions
For those who want to practice coding while reading, we recommend using the online JavaScript Playground linked below. Enjoy coding!
Online JavaScript Playground:
Try experimenting with your code using this interactive platform.
Section 1.1: Checking if a String is Empty
Question 1: How can we determine if a string is empty?
Answer: It's important to understand the methods we use, such as the .trim() method, which eliminates whitespace from both ends of a string and returns a new string without altering the original.
function isEmptyString(str) {
return str.trim().length === 0;
}
console.log(isEmptyString("")); // true
console.log(isEmptyString("Hello")); // false
console.log(isEmptyString(" ")); // true (trimmed length is 0)
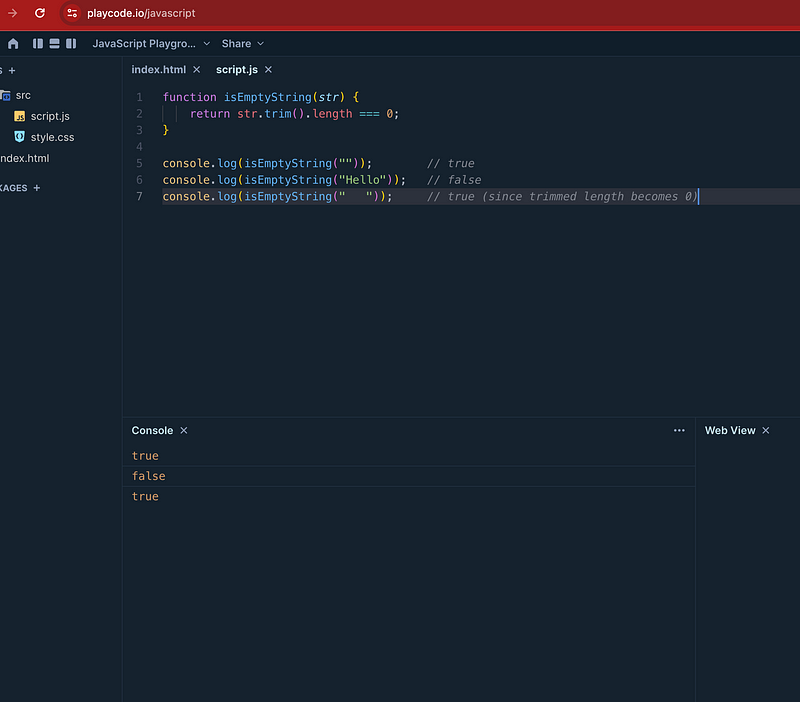
Without Trim Output:
function isEmptyString(str) {
return str.length === 0;
}
console.log(isEmptyString("")); // true
console.log(isEmptyString("Hello")); // false
console.log(isEmptyString(" ")); // false (no trimming)
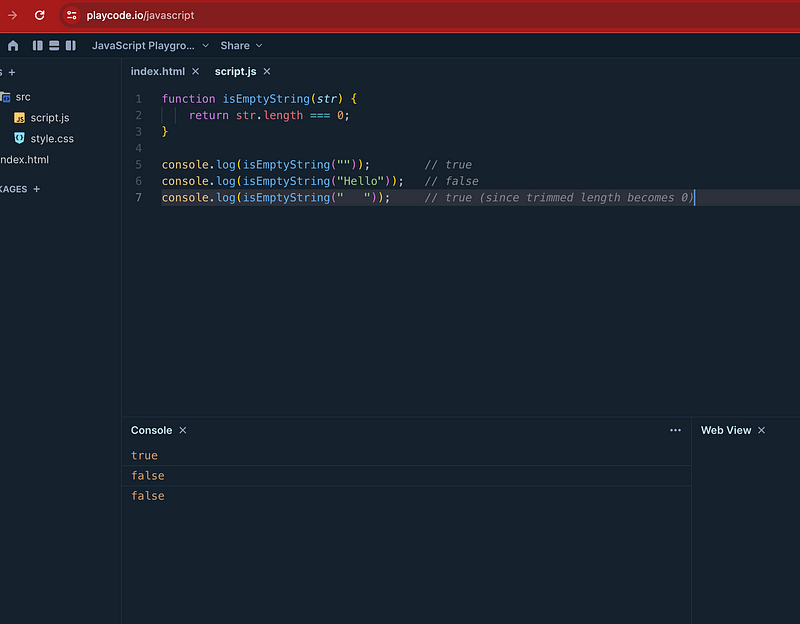
Tip: Explore trimEnd() and trimStart() to see how they operate differently.
Section 1.2: Removing Whitespace Characters
Question 2: How do we eliminate all whitespace characters from a string?
Answer:
Method 1 — Using Regular Expressions:
const inputString = 'hello ntHow are you?';
console.log(inputString.replace(/s/g, ''));
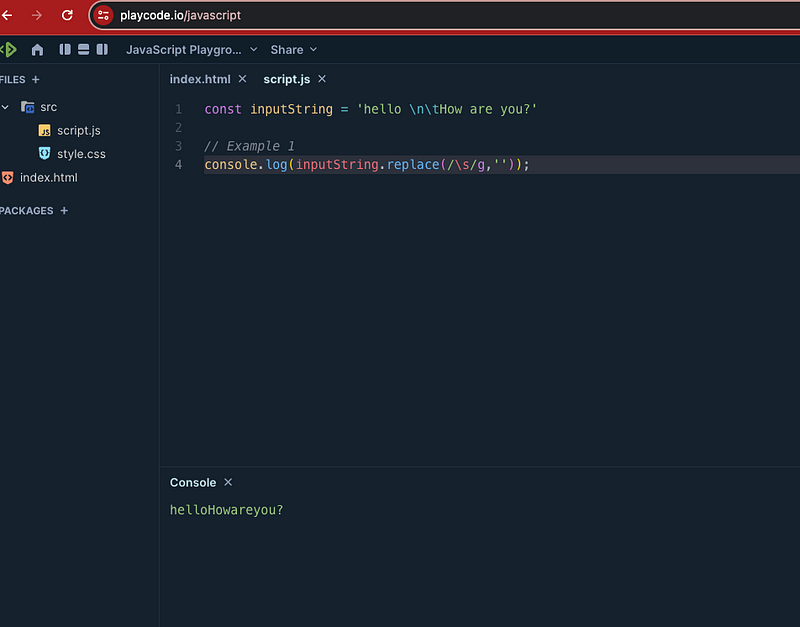
Method 2 — Using Split and Join:
console.log(inputString.split(/s+/).join(''));
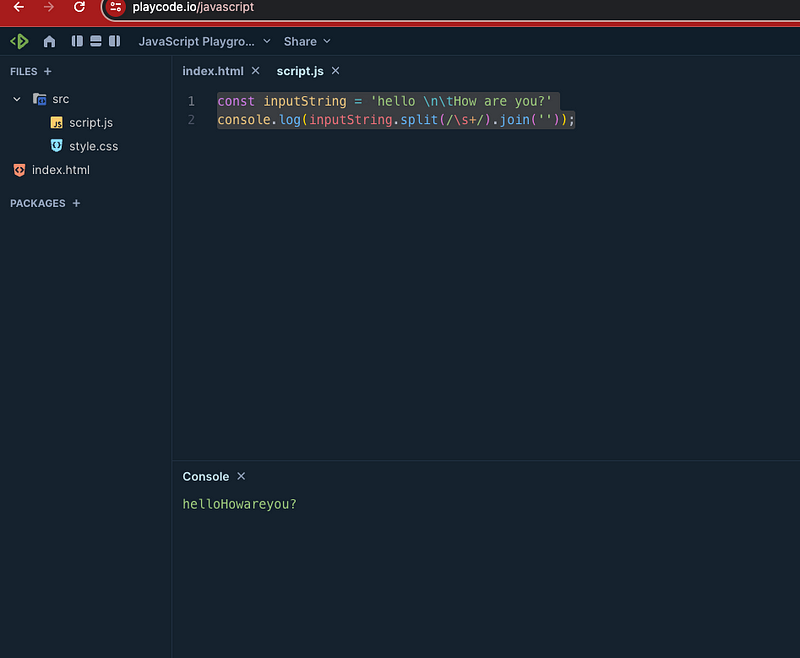
Section 1.3: Counting Vowels in a String
Question 3: How can we count the number of vowels in a given string?
Answer:
Method 1 — Simple For Loop:
const sentence = "my best world is my home only";
const vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U'];
let count = 0;
for (let character of sentence) {
if (vowels.includes(character)) {
count++;}
}
console.log(count);
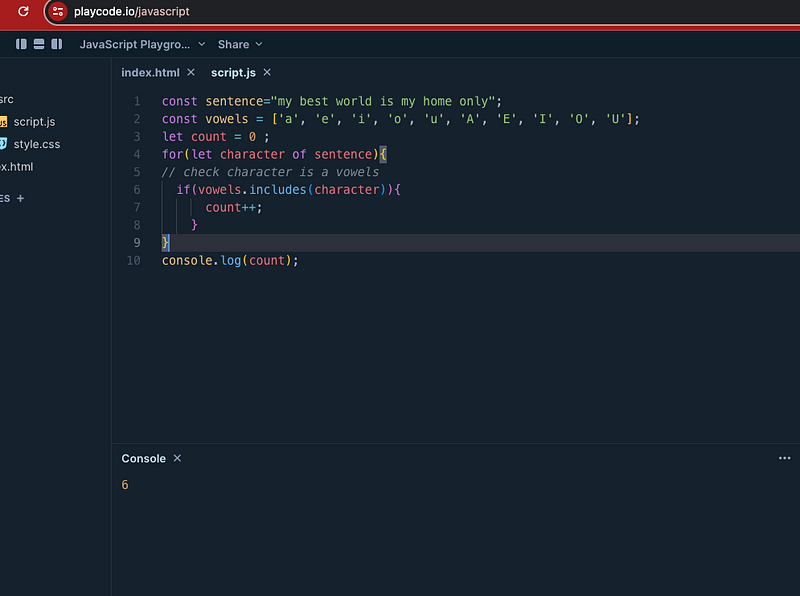
Method 2 — Using Regular Expressions:
const vowelRegex = /[aeiou]/gi;
const matches = mybestword.match(vowelRegex);
const count = matches ? matches.length : 0;
console.log(count);
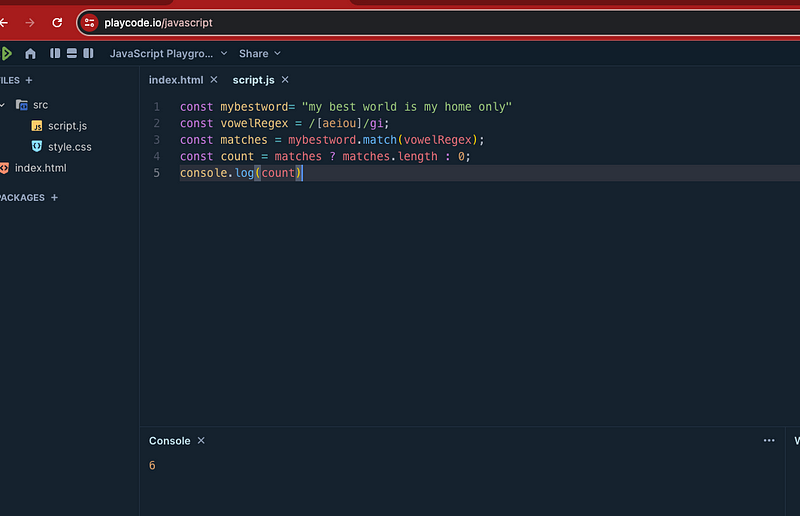
Section 1.4: Truncating a String
Question 4: How can we truncate a string if it's longer than a specified maximum length?
Answer:
const sentence = "my world is my home";
const maxLength = 10;
const truncated = sentence.length > maxLength ? sentence.slice(0, maxLength) + '..' : sentence;
console.log(truncated); // truncated string
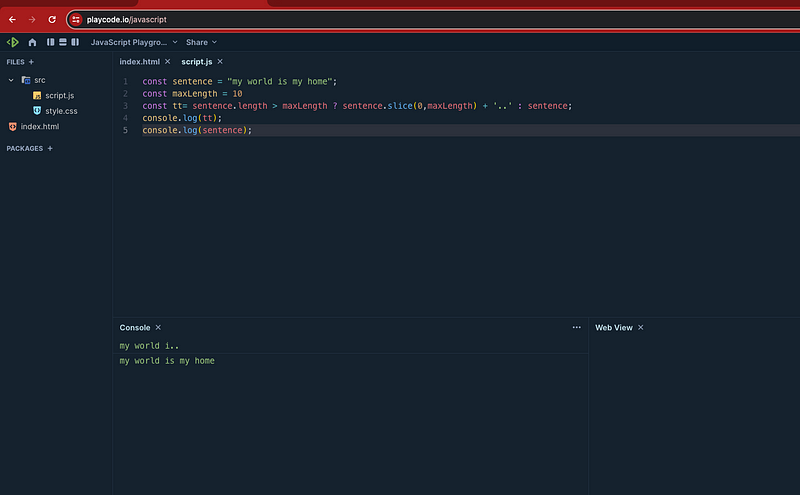
Section 1.5: Removing Duplicate Words
Question 5: How can we eliminate duplicate words from sentences?
Answer: We invite all our coding friends to share their solutions in the comments!
We will continue our exploration with arrays and JavaScript data types in the upcoming week, from April 1st to April 7th, 2024.
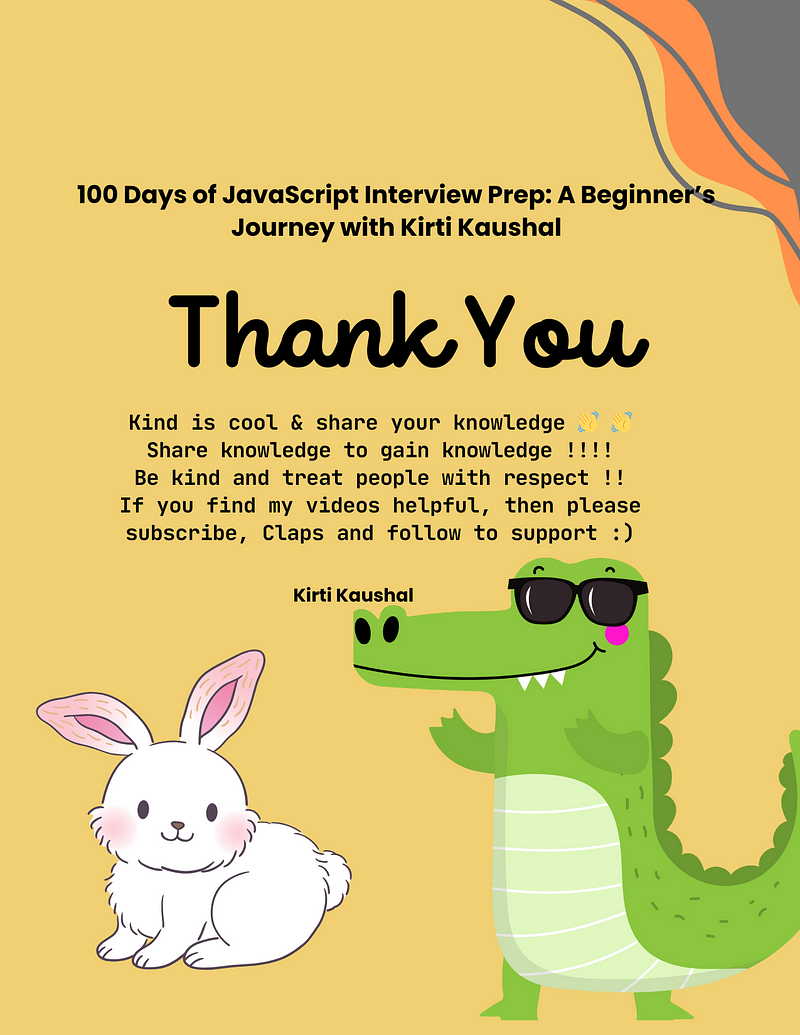
Stay tuned for more, and happy coding! Remember, kindness is key—share knowledge to foster growth!
If you find this content helpful, consider subscribing and supporting the channel.
Mastering JavaScript - EVERYTHING You Need To Know - YouTube
Explore the essential concepts of JavaScript in this comprehensive guide.
Introduction to Javascript | Complete Course 2024 | Zero to Advanced in 6 hours - YouTube
A complete course designed to take you from beginner to advanced in JavaScript.