Implementing bcrypt.js for Secure Password Hashing in Node.js
Written on
Chapter 1: Introduction to bcrypt.js
In this chapter, we will explore how to integrate the bcrypt.js library into your Node.js projects for secure password hashing.
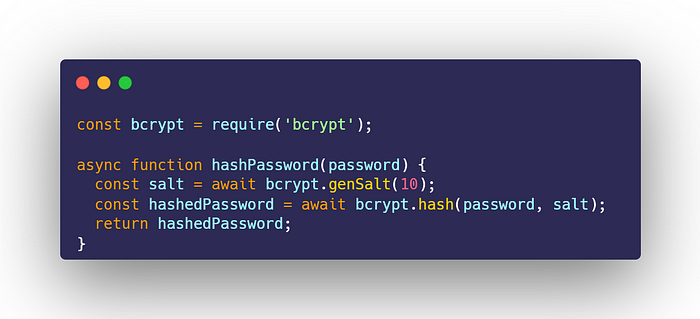
To begin, the first step is to install the bcrypt library:
- Install the library:
npm install bcrypt
After that, you will need to import the library into your code:
const bcrypt = require('bcrypt');
Next, we will create an asynchronous function to hash passwords:
async function hashPassword(password) {
const salt = await bcrypt.genSalt(10);
const hashedPassword = await bcrypt.hash(password, salt);
return hashedPassword;
}
Let’s break down this function step-by-step:
- Importing bcrypt: The line const bcrypt = require('bcrypt'); loads the bcrypt library into your Node.js application. The require() function is essential for importing external modules.
- Defining the hashPassword function: The line async function hashPassword(password) { declares an asynchronous function named hashPassword. The async keyword signifies that this function will perform operations that may take time to finish. It accepts one argument: password, which represents the plain-text password to be hashed.
- Generating a salt: The command const salt = await bcrypt.genSalt(10); generates a random salt using bcrypt.genSalt(). The await keyword indicates that the function should pause until the salt is generated. The number 10 denotes the cost factor, impacting the security and speed of the hashing process. A higher number results in stronger security but slower performance.
- Hashing the password: The line const hashedPassword = await bcrypt.hash(password, salt); hashes the password using the generated salt. Again, the await keyword is utilized because hash() is an asynchronous operation. This function outputs the hashed password, which is stored in the hashedPassword variable.
- Returning the hashed password: Finally, the line return hashedPassword; sends back the hashed password from the function, allowing it to be stored in a database or used for authentication purposes.
Endnotes: It is crucial to choose a strong cost factor (like 10 or higher) to enhance the security of your hashed passwords. Ensure that the generated salt is securely stored and consistently used for hashing and verifying passwords.
Chapter 2: Video Tutorials on Password Hashing
To further illustrate the process of password hashing in Node.js, we recommend watching the following videos:
The first video, "Hashing Passwords in Node and Express using bcrypt," offers a practical demonstration of how to use bcrypt for password hashing.
The second video, "Password Hashing in Node.js with bcrypt," provides additional insights and techniques for implementing bcrypt in your applications.