Getting the Top Position of an Element Relative to the Viewport
Written on
Understanding the Element's Top Position in the Viewport
At times, you may need to determine the top position of an element in relation to the browser's viewport. This guide explores methods to achieve that.
Using the getBoundingClientRect Method
One effective way to ascertain an element's position is by utilizing the getBoundingClientRect method. This function provides information about an element's dimensions and its position relative to the viewport.
For example, you can use the following code:
const div = document.querySelector('div');
const { top: t, left: l } = div.getBoundingClientRect();
console.log(t, l);
In this snippet, we select a div element with querySelector. The getBoundingClientRect method returns an object that contains the top and left properties. Here, top indicates the distance from the top of the viewport, while left indicates the distance from the left side, both measured in pixels.
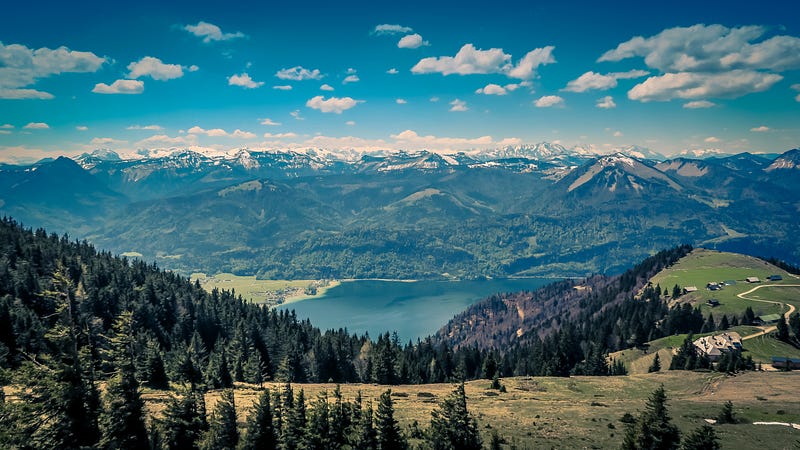
If scrolling is a factor, you can incorporate the scrollY and scrollX values to adjust the element's position. The revised code would look like this:
const div = document.querySelector('div');
const { top: t, left: l } = div.getBoundingClientRect();
const { scrollX, scrollY } = window;
const topPos = t + scrollY;
const leftPos = l + scrollX;
console.log(topPos, leftPos);
This adjustment allows you to account for any scrolling that may affect the element's position.
The first video, "Translating Viewport Coordinates Into Element-Local Coordinates Using .getBoundingClientRect()", delves deeper into the getBoundingClientRect method and its applications.
Conclusion
In summary, the getBoundingClientRect method is a valuable tool for determining an element's position relative to the viewport. By following the techniques outlined, you can effectively find the positioning you need.
The second video, "Center an Element within the #Viewport with #Position and #Transform #Translate," provides insights on positioning elements within the viewport using CSS techniques.